useCopilotChatSuggestions
A hook for providing suggestions to the user in the Copilot chat.
useCopilotChatSuggestions is experimental. The interface is not final and can change without further notice.
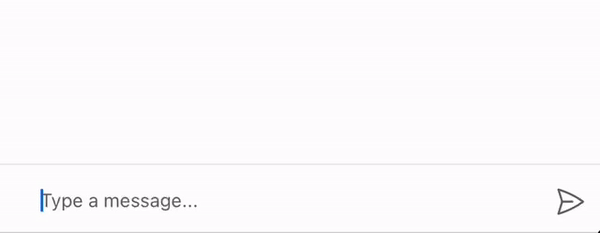

useCopilotChatSuggestions
integrates auto-generated chat suggestions into your application in the context of your
app’s state. It dynamically manages suggestions based on provided configurations and
dependencies.
Basic Setup
To incorporate this hook into your React components, start by importing it:
import { useCopilotChatSuggestions } from "@copilotkit/react-ui";
Then, use it in your component to initiate suggestion functionality:
useCopilotChatSuggestions({
instructions: "Your instructions for suggestions.",
});
Dependency Management
import { useCopilotChatSuggestions } from "@copilotkit/react-ui";
useCopilotChatSuggestions(
{
instructions: "Suggest the most relevant next actions.",
},
[appState],
);
In the example above, the suggestions are generated based on the given instructions.
The hook monitors appState
, and updates suggestions accordingly whenever it changes.
Behavior and Lifecycle
The hook registers the configuration with the chat context upon component mount and removes it on unmount, ensuring a clean and efficient lifecycle management.
Parameters
A prompt or instructions for the GPT to generate suggestions.
The minimum number of suggestions to generate. Defaults to 1
.
The maximum number of suggestions to generate. Defaults to 3
.
An optional class name to apply to the suggestions.