Copilot Textarea
Learn how to use the Copilot Textarea for AI-powered autosuggestions.
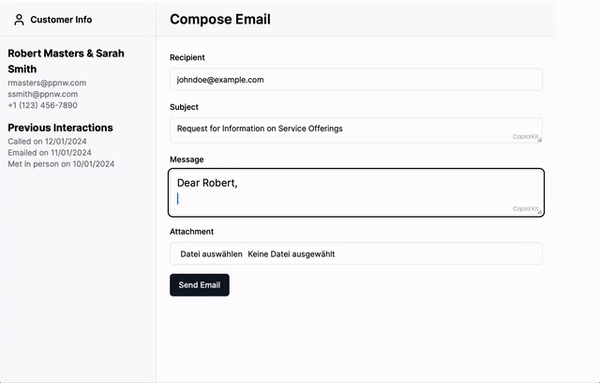
<CopilotTextarea>
is a React component that acts as a drop-in replacement for the standard <textarea>
,
offering enhanced autocomplete features powered by AI. It is context-aware, integrating seamlessly with the
useCopilotReadable
hook to provide intelligent suggestions based on the application context.
In addition, it provides a hovering editor window (available by default via Cmd + K
on Mac and Ctrl + K
on Windows) that allows the user to
suggest changes to the text, for example providing a summary or rephrasing the text.
This guide assumes you have completed the quickstart and have successfully set up CopilotKit.
Add CopilotTextarea
to Your Component
Below you can find several examples showing how to use the CopilotTextarea
component in your application.
Next Steps
- We highly recommend that you check out our simple Copilot Textarea Tutorial.
- Check out the full CopilotTextarea reference